Lab 2: iSwat Media Library
Due on Sunday, September 18th at 11:59 PM. This is a team lab. You and your assigned lab partner(s) will complete this lab together. Make sure that you are familiar with the Partner Etiquette guidelines. You may discuss the concepts of this lab with other classmates, but you may not share your code with anyone other than course staff and your lab partner(s). Do not look at solutions written by students other than your team. If your team needs help, please post on the Piazza forum or contact the instructor or ninjas. If you have any doubts about what is okay and what is not, it’s much safer to ask than to risk violating the Academic Integrity Policy.
Overview
In this lab, you will write a small application for managing a library of multimedia files. You will:
- Make use of polymorphism and inheritance
- Gain further experience with C++
- Work with your lab partner to develop a piece of software
Because this is a team lab, you and your partner will share a repository. The
URL for your repository will be git@github.swarthmore.edu:cs35-f16/lab02-<your-teamname>
.
You should have received an e-mail informing you of your team name. You can
also find your team’s repository by visiting
the GitHub organization for this class.
iSwat Media Library

Your task will be to develop a command-line program to manage media files. In
particular, we are concerned with images, text, and online video clips in this
assignment. You will write three classes – Image
, Text
, and Video
–
which extend a base class Media
and implement common behavior. You will then
implement a MediaLibrary
class which will read information from a media
library file and allow the user to list or open the media contained within.
Starting Code
Your repository should contain the following starter files. Those which you may need to change appear in bold.
media.h
: The declaration of the abstractMedia
class.text.h
/text.cpp
: TheText
class that you will implement.image.h
/image.cpp
: TheImage
class that you will implement.video.h
/video.cpp
: TheVideo
class that you will implement.mediaLibrary.h
/mediaLibrary.cpp
: TheMediaLibrary
class that you will use to keep track of yourMedia
objects.main.cpp
: Themain
function for your program.manualTests.cpp
: Anothermain
method that you can use to experiment with your code.Makefile
: The script that builds your code for you.
The Media
Class
The Media
class contains virtual
methods which are common to all media. You
will implement each of these methods in the Image
, Text
, and Video
subclasses. See the method comments on the declarations of those classes for
information about the behavior you need to implement.
The MediaLibrary
Class
This class keeps track of the Media
objects in a library. It allows a caller
to ask how many Media
objects there are and gives access to them upon request.
Its constructor will read a media library file and create the Media
objects
accordingly.
The Media Library File

When the program starts, it must be given a media library file as its only command-line argument, like so:
./iswatmedia test.library
Your MediaLibrary
constructor will need to read the contents of this file and
create an appropriate collection of Media
objects for it. The first line of
the file indicates the number of media items in the file. Each item is
represented by several lines depending upon what kind of media it is:
- For a text file, the next line will be
text
. The following line will contain the name of the text file (e.g./usr/local/doc/GettysburgAddress
); the next line will contain the document’s title (e.g.Gettysburg Address
). - For an image, the next line will be
image
. The following line will contain the name of the image file (e.g./usr/swat/pics/brownflames.gif
); the next line will contain the image’s title (e.g.Brown Flames
). - For a video clip, the next line will be
video
. The following line will be the URL for the video (e.g.https://vimeo.com/28241047
). The line after that will be the title of the video (e.g.Running Gerbil
). The next line after that will be the video length as a string (e.g.00:29
).
For instance, the following is a valid media library file:
3
image
/usr/swat/pics/brownflames.gif
Brown Flames
image
/usr/swat/pics/aliens.jpg
Aliens
text
/usr/local/doc/GettysburgAddress
Gettysburg Address
The Application
As mentioned above, your main
function should take the name of a media
library file as its only argument. Using that file, it should display a menu
listing the media files (in order) and allow the user to pick a media file to
open. Media files should be listed by positive integers (that is, the first
media file you show should be selected by the input 1
). After opening that
media file, the menu should be repeated again; the program should continue in
this fashion until zero is entered, after which it should terminate.
To display the media in a menu, you need to have a way of representing it. The
describe
method is virtual
and unimplemented; each Media
subclass
implements it in a different way.
- The
getDescription
method onText
should give the string"[text] "
followed by the name of the document (e.g."[text] Gettysburg Address"
). - The
getDescription
method onImage
should do likewise but with the prefix"[image]"
(e.g."[image] Brown Flames"
). - The
getDescription
method onVideo
uses the prefix"[video]"
and suffixes the video length in parentheses (e.g."[video] Running Gerbil (00:29)"
).
The act of opening a media file is also performed by the particular Media
subclass. The open
method of each Media
object should behave as follows:
- The
open
method onText
should open the file, read it line by line, and print each line it reads. - The
open
method onImage
should open the image viewergpicview
to display the image to the user. You can accomplish this by using thesystem
function discussed below. - The
open
method onVideo
should open the URL of the video usingfirefox
. You should usesystem
for this as well.
The system
Function

The library cstdlib
contains a function system
which can run commands for
you. You pass a C-style string to the system
function and it will run it in
much the same way as the shell we use to e.g. compile our programs. For
instance, the following program will open Firefox to the Google home page.
After it does that, it will open a text editor.
#include <cstdlib>
#include <string>
using namespace std;
int main(int argc, char** argv) {
string cmd1 = "firefox http://www.google.com";
string cmd2 = "gedit";
system(cmd1.c_str());
system(cmd2.c_str());
return 0;
}
In larger applications, we avoid using the system
function in favor of more
precise ways to launch other programs from within our own code. For this lab,
however, the system
function suits us quite well.
Invalid Input
In this lab, you are not permitted to assume that the user gives valid input. The user may mistype the name of the media library file, for instance, or give a string instead of a number when you ask which file to open. Your program must handle these situations gracefully. If the user gives a string rather than a number, for instance, you should print an appropriate error message and then show the menu again. If the media library file does not exist, you should print a message and then quit (with a non-zero exit code, if you like).
To deal with the user typing an incorrect input for the menu, try reading the
entire line that the user inputs and then converting that line into a string
using the stoi
function. You can then catch any exception that the stoi
function raises, like so:
string inputLine;
getline(cin, inputLine);
try {
int choice = stoi(inputLine);
// If you reach this line, then everything is fine.
} catch (exception& e) {
// If you reach this line, then something went wrong!
}
// You will reach this line either way.
Exceptions in C++ work much like they do in Python and Java.
You are permitted to assume that, if a media library file exists, it is formatted correctly; you do not have to worry about e.g. the file being empty or containing a non-integer on the first line.
Getting Started
Remember: you don’t have to write every program all at once. Consider using the following approach to completing your lab assignment:
-
Complete the code for the
Text
class first. A little bit more of that code has been provided for you. -
A file
manualTests.cpp
exists for your convenience. In that file, write some code that creates aText
object and calls bothgetDescription
andopen
. Make sure it does what you think it should do. Until it does, don’t write new code. Get theText
class to work correctly before continuing on to the rest of the lab. -
Based on your work on
Text
, implement theImage
andVideo
classes. They will be similar, but not identical. -
Next, write your
MediaLibrary
class. Again, try writing a few short snippets of code to see if it works. -
Finally, write your
main
function to construct theMediaLibrary
object, present a menu, and open media files until the user decides to quit.
The approach described above – writing a little bit of your program and then writing some tests to see if things work correctly – is a powerful skill. By testing pieces of your code in isolation, you gain confidence in your implementation and it becomes easier to track down bugs and, at a higher level, understand what your code is doing.
Coding Style Requirements
You are required to observe some good coding practices:
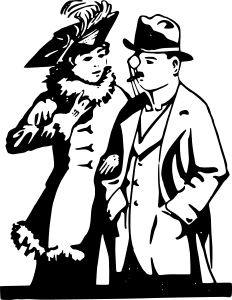
-
You should pick meaningful variable names.
// Good int* pixels = new int[size]; // Bad int* p = new int[size];
-
You should use correct and consistent indentation. Lines of code within a block (that is, surrounded by
{
and}
) should be indented four spaces further than the lines surrounding them.// Good if (condition) { cout << "Test" << endl; } // Bad if (condition) { cout << "Test" << endl; }
-
You should use a block whenever possible, even if it’s not necessary. This helps you avoid subtle or messy bugs in the future.
// Good if (condition) { cout << "Something" << endl; } // Bad if (condition) cout << "Something" << endl;
Peer Review
As this is a team assignment, you are required to complete a peer review of your lab partner or partners. You must do this even if everything is fine. You should complete this peer review after you have turned in your assignment. The peer review comes in the form of a simple Google Form and can be accessed here; in most cases, it will likely take less than a minute to complete. If you have trouble accessing this peer review, please make sure that you are logged into Google using your Swarthmore credentials.
Your peer review will be kept private; only course staff will have access to this information. You can also update your peer review after you have submitted it. However, if you do not submit a peer review, you will lose participation grade points. Please don’t forget!
Summary of Requirements
Your program should
- Have a different
Media
subclass for each of the three kinds of media - Use the
MediaLibrary
constructor to read a media library file and create appropriateMedia
objects - Use polymorphism to address how each different type of
Media
object is opened - Use the
system
function to launch helper programs as described above - Validate user input and respond appropriately if invalid input is given
Example Execution
Here’s an example run of the program:
$ ./iswatmedia example1.library
1. [image] Brown Flames
2. [image] Aliens
3. [text] Gettysburg Address
0. (quit)
1
1. [image] Brown Flames
2. [image] Aliens
3. [text] Gettysburg Address
0. (quit)
3
Four Score and seven years ago our fathers brought forth on
this continent a new nation, conceived in Liberty, and dedicated
to the proposition that all men are created equal.
Now we are engaged in a great civil war, testing whether that
nation, or any nation so conceived and so dedicated, can long endure.
We are met on a great battle-field of that war. We are met to dedicate
a portion of it as the final-resting place of those who here gave
their lives that that nation might live. It is altogether fitting
and proper that we should do this.
But in a larger sense we cannot dedicate, we cannot consecrate, we
cannot hallow this ground. The brave men, living and dead, who
struggled here, have consecrated it far above our power to add or
detract. The world will little note nor long remember what we say
here, but it can never forget what they did here. It is for us, the
living, rather to be dedicated here to the unfinished work that they
have thus far so nobly carried on. It is rather for us to be here
dedicated to the great task remaining before us, --that from these
honored dead we take increased devotion to the cause for which they
here gave the last full measure of devotion, --that we here highly
resolve that the dead shall not have died in vain, that the nation
shall, under God, have a new birth of freedom, and that the
government of the people, by the people, and for the people, shall
not perish from the earth.
1. [image] Brown Flames
2. [image] Aliens
3. [text] Gettysburg Address
0. (quit)
-1
Invalid entry.
1. [image] Brown Flames
2. [image] Aliens
3. [text] Gettysburg Address
0. (quit)
brown flames
Invalid entry.
1. [image] Brown Flames
2. [image] Aliens
3. [text] Gettysburg Address
0. (quit)
0
Goodbye!
$ ./iswatmedia eggsample1.library
Your media library is either empty or invalid.
$